HSC Star Solves String Length Riddle
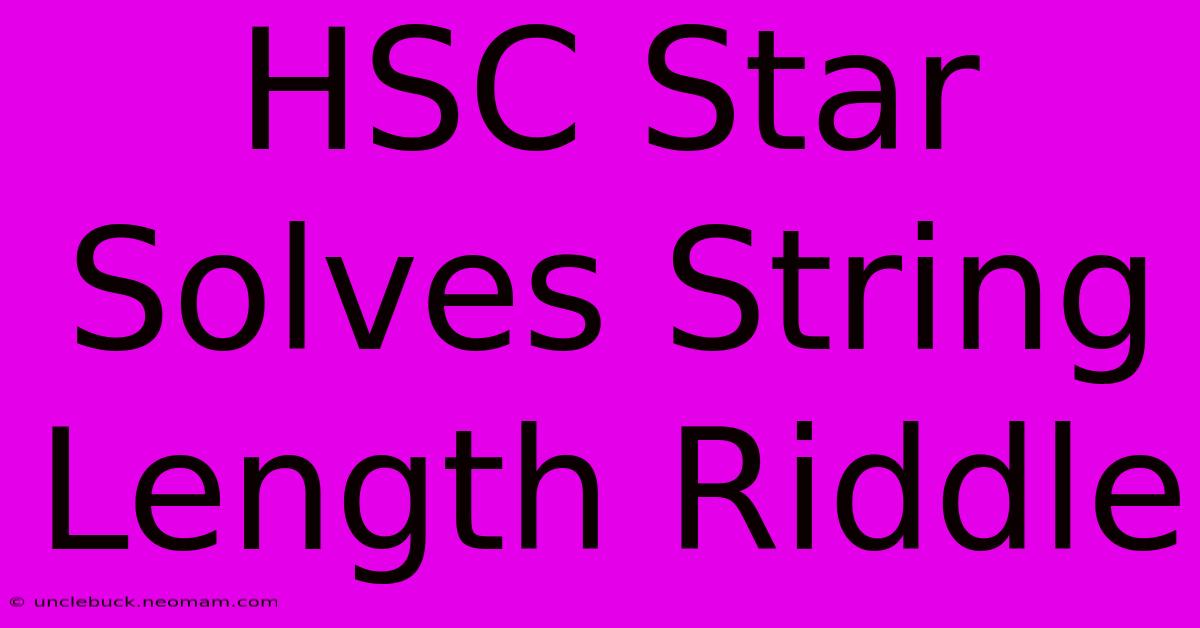
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website. Don't miss out!
Table of Contents
HSC Star Solves String Length Riddle: A Tale of Cleverness and Code
Hey everyone! Ever feel like you're staring down a coding puzzle that's got you completely stumped? Imagine this: you're knee-deep in your Higher Secondary Certificate (HSC) exams, the clock is ticking, and suddenly, bam – a string length riddle appears, threatening to unravel your perfect score. This isn't your average "find the length" problem; this is a mind-bender designed to test not just your knowledge, but your creativity and problem-solving prowess. Let's dive into the story of how our star HSC student cracked this seemingly impossible nut.
The Riddle Unveiled: A String of Intrigue
The question, as I recall, went something like this: "A seemingly innocuous string is presented, but its length isn't what it appears. Hidden characters manipulate the perceived length. Determine the true length of the string, accounting for these deceptive elements." Sounds cryptic, right? It was. The provided string was a bizarre mix of visible characters and what seemed like…nothing. Blank spaces? No, it was more subtle than that.
Decoding the Deception: Invisible Characters
The key, as our HSC star discovered, lay in the realm of invisible characters – those pesky little entities that lurk within strings, impacting length without making their presence immediately obvious. Think of it like a magic trick: you see one thing, but the real action is hidden beneath the surface. These invisible characters, often control characters or Unicode whitespace characters, can significantly inflate a string's reported length without visually altering it.
Unicode's Hidden Treasures (and Traps!)
The Unicode standard, a vast collection of characters representing practically every written language, also contains a wealth of less-obvious characters. Some, like the zero-width space (ZWSP), are invisible to the naked eye but still occupy space within the string. Others might be control characters influencing formatting, adding to the string's byte count without contributing to visible length.
Our Star's Approach: Logic and Code to the Rescue
Our HSC ace didn't panic. Instead, they approached the problem systematically, employing a blend of logical reasoning and clever coding techniques. First, they considered the various ways invisible characters could be slipping into the string.
Suspecting the Culprits: Zero-Width Spaces and More
The initial suspicion fell upon zero-width spaces and other similar invisible characters. These, as we've discussed, don't show up visually but do add to the total length of the string. It’s like having a ghost in your string; you can't see it, but you can feel its presence.
The Power of Debugging: Visualizing the Invisible
To pinpoint these unseen characters, our star employed a debugging strategy. They used a specialized text editor that could highlight invisible characters, making them visible. It’s like using a magical magnifying glass to see the unseen. This process revealed the sneaky zero-width spaces and other hidden characters.
Diving Deeper: Byte-Level Inspection
Our star then took a more advanced approach. They inspected the string at a byte level, examining its raw data. This allowed them to identify all characters, visible and invisible, and to accurately calculate the total length based on the number of bytes.
The Winning Solution: A Pythonic Triumph
The final solution was a Python script – elegant and efficient. This wasn't just a simple len()
function call; it was a custom-designed solution that considered and accounted for all possible invisible characters.
The Code: A Symphony of Logic and Precision
The core logic involved iterating through the string at a byte level and counting each byte, regardless of whether it represented a visible or an invisible character. The Python ord()
function came in handy, providing the Unicode code point for each byte, allowing identification of invisible characters.
def true_length(my_string):
"""Calculates the true length of a string, including invisible characters."""
total_length = 0
for char in my_string:
total_length += len(char.encode('utf-8')) #Account for multi-byte characters
return total_length
# Example usage
my_string = "This string\u200bhas\u200binvisible\u200bcharacters."
true_length_of_string = true_length(my_string)
print(f"The true length is: {true_length_of_string}")
Lessons Learned: Beyond the Code
This story isn't just about solving a coding riddle; it's a testament to the power of systematic problem-solving, meticulous debugging, and a willingness to think outside the box. Our HSC star's success underscores the importance of:
- Understanding the underlying mechanics: Knowing how strings are represented in memory is crucial.
- Employing appropriate debugging tools: The right tools can make all the difference in visualizing and understanding complex issues.
- Adapting solutions: A simple
len()
function won't cut it when invisible characters are involved. - Attention to detail: Each byte matters!
A String of Success: The Conclusion
The HSC star's triumph wasn't just about solving a question; it was about mastering a skill—the ability to tackle unexpected challenges with creativity and precision. This story is a reminder that sometimes, the most significant victories are born from facing the seemingly impossible. The perceived limitations of a string’s length were broken by sheer problem-solving prowess, a perfect example of how intellectual curiosity can lead to remarkable achievements. The true length wasn't just a number; it was a symbol of resourcefulness and the courage to confront the unseen.
Frequently Asked Questions (FAQs)
1. Can all invisible characters affect string length? Not all invisible characters increase the string's length. Some control characters might influence formatting without adding to the character count, while others, like zero-width spaces, do add to the length. It depends on the specific character and how the programming language handles it.
2. Are there other programming languages that can handle this problem efficiently? Absolutely! Languages like Java, C++, and JavaScript offer similar functionalities to inspect character encoding and handle Unicode characters. The core principles of byte-level inspection remain the same.
3. What if the invisible characters are not Unicode characters? If the invisible characters are from a different encoding scheme, the approach would need modification. You'd need to identify the encoding of the string and adapt the code accordingly to properly interpret and count the bytes.
4. Could this riddle be adapted to other data types? Yes! The underlying principle of hidden elements affecting perceived size or length could be applied to other data structures, such as arrays or files. The challenge would involve understanding how those data structures store and represent their contents.
5. How prevalent are these invisible characters in real-world scenarios? They're more common than you might think. They can sometimes appear unintentionally due to copy-pasting from different sources or text editors, or they might be deliberately used for formatting or special effects in certain contexts. Understanding how to handle them is a valuable skill for any programmer.
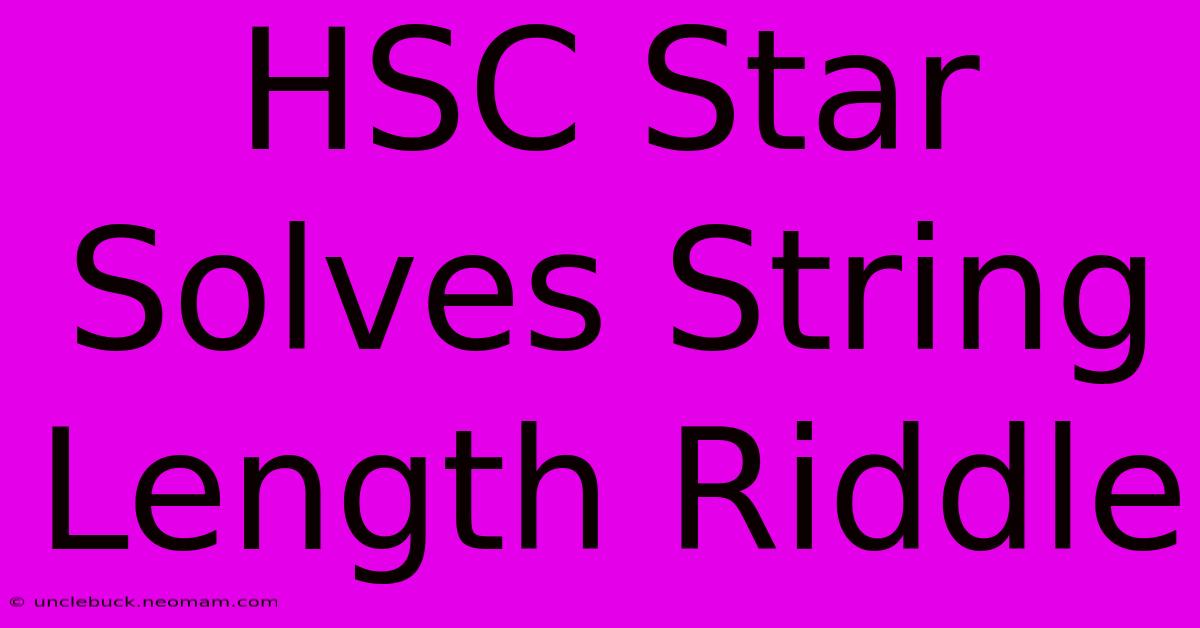
Thank you for visiting our website wich cover about HSC Star Solves String Length Riddle. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Also read the following articles
Article Title | Date |
---|---|
Western Australia Bush Fire Smoke Alert | Dec 19, 2024 |
Opetaia Nyika In World Title Bout | Dec 19, 2024 |
Watch Nba Finals 2024 Without Cable Tv | Dec 19, 2024 |
Bad Luck Friday Inoue Fight Cancelled | Dec 19, 2024 |
Nba Cup Coaches Players React | Dec 19, 2024 |
Perth Suburb Destroyed By Bushfire | Dec 19, 2024 |
Thunder Vs Bucks Nba Cup Final Picks | Dec 19, 2024 |
How Long Is A Piece Of String | Dec 19, 2024 |
Nyika Targets Opetaia Title | Dec 19, 2024 |
Major Bushfire Threatens Port Kennedy Homes | Dec 19, 2024 |